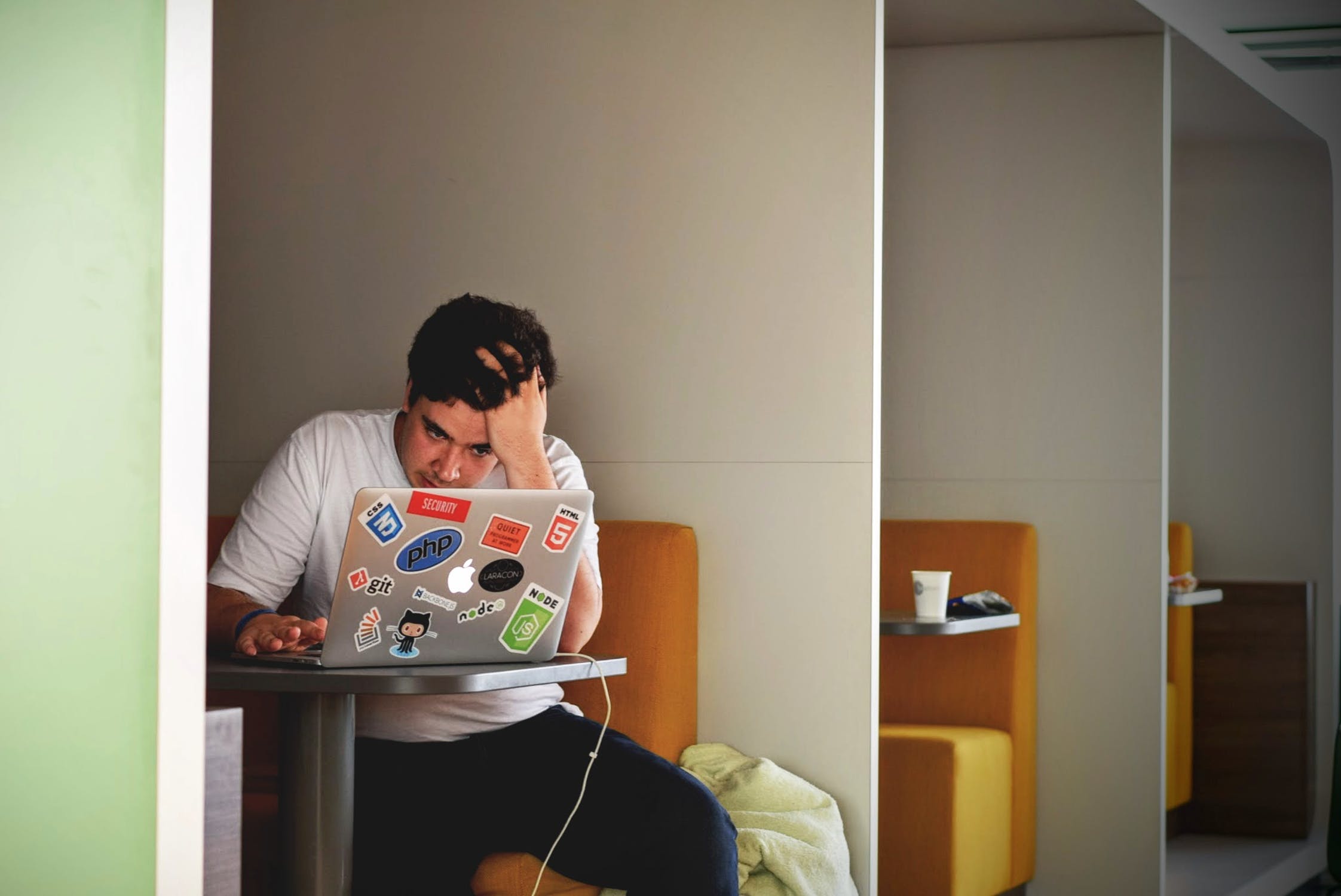
PHP vs NODEJS
Hey all, I wanted to write something about this for a long time
As you guys know, I’ve been coding robust and complex applications using Laravel, which is one of the best MVC framework out there, but I have plenty of experience in multiple languages like Action Script (Macromedia Flash haha), Java, JavaScript, PHP and during the past few years I’ve seen all this hype about NODEJS as a backend, so I wanted to get my hands dirty on it, here’s my opinion about the topic!
The Basics
What is php?
PHP means (Hypertext Preprocessor) this code executes on the server and generates HTML, its quite simple to get started for new developers.
PHP pages contain HTML with embedded code that does “something” (in this case, output “Hi, I’m a PHP script!”).
<!DOCTYPE html> <html> <head> <title>Example</title> </head> <body> <?php echo "Hi, I'm a PHP script!"; ?> </body> </html>
Simple right? Awesome!
What is MVC?
Once you start coding if you are not organized you will start to make mistakes like adding logic to where you display messages to users and after a while it just a complete mess. MVC stand for Model, View, Controller.
Model: Basically, you can create classes that will be interpret as tables in your DB (ORM like eloquent), all logic is created here, meaning that you create a Car Class, and within your application you can perform:
$car = Car::get(1); $car->drivers(); // get list of drivers $car->mileage; // get the mileage of an specific car.
Controller: A user goes to your https://website.com/dashboard and here they expect to have the analytics or the info of their account in your application, here you need to retrieve the data from the DB, probably make some calculations, and then send the render data to your View.
View: Here is where your HTML is displayed. You get an array, json or any other datatype sent from the controller and display it on this HTML view.
The MVC framework makes your application super easy to distribute in a logic way and lets you change a process, a view or a model without compromising the rest of your application.
What is Laravel?
So Laravel is one of the most popular MVC framework for php, I love it and its great to use on a big team, and since its super clear and lots of things out of the box then its SUPER easy to get started. You can learn more here.
What is NodeJS
Google designed an engine to run JavaScript on their fast browser, Google Chrome. It turns out that Ryan Dahl basically took that engine and created a runtime so you can execute JavaScript outside the client’s browser and run it on a computer/server. This changed the course of web development forever.
NodeJS has quickly adapted because frontend developers that were executing code on the client side, they are now able to execute code on server side, building full stack applications with a single programming language!
If you want send me an email, if you want me to expand on NodeJS or their MVC Framework ExpressJS!
PHP and NodeJS Differences:
For me the main difference if that PHP is synchronous and NodeJS is Asynchronous. What is that?
Let’s imagine we are at a restaurant with 6 tables, 1 waitress and one chef.
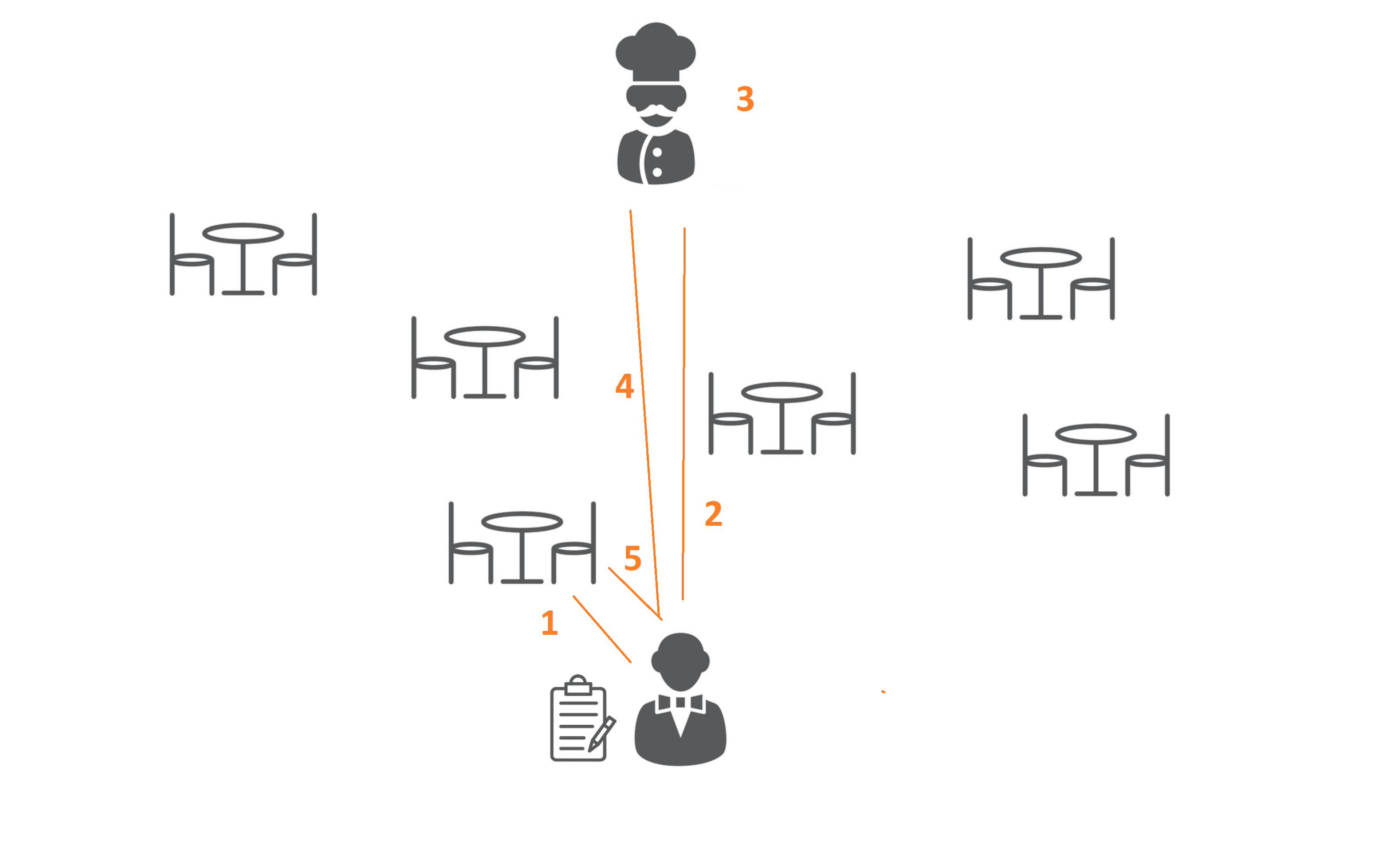
Synchronous:
- Waitress come and take your order
- Proceed to take this order to the chef
- He WAITS for the Chef to cook the meal. Once ready
- Waitress takes the cooked meal to the client’s table
- (not pictured) He is ready to take orders again.
Easy right? Now. What happens if 2,3,4,5,6 people come and each seat in all the remaining seats? They will only be served when client 1 has its food. The client #6 will have to wait until everyone is served before, he even orders the food!! That means $0 tip for our waitress.
In this scenario Asynchronous are much like real life:
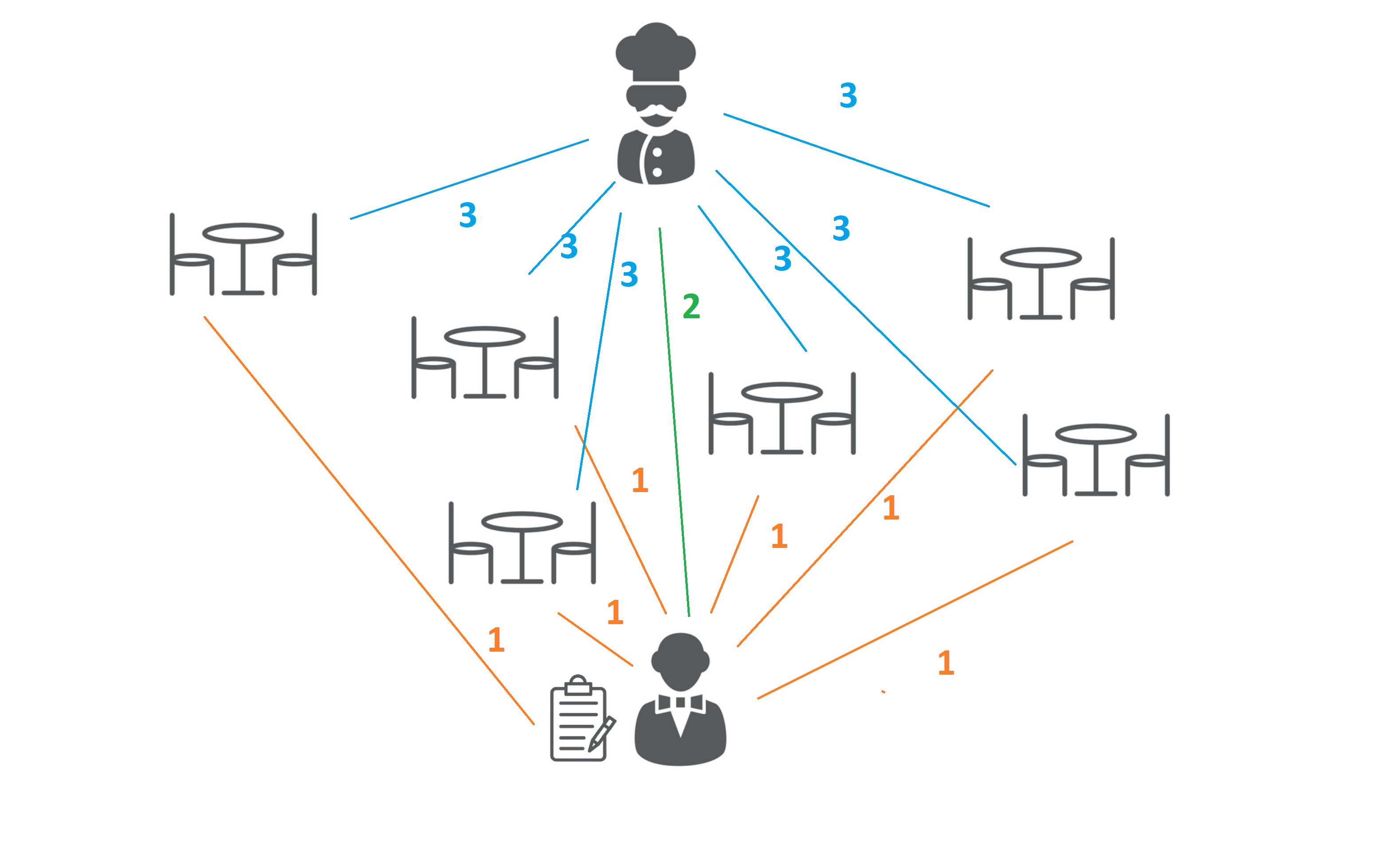
Asynchronous
- Waitress get orders as soon as clients arrives and deliver to kitchen
- Waitress deliver the order to the kitchens after it was requested and LEAVE
- Waitress is chilling or taking orders until food is ready.
That’s the magic of ASYNC requests. You leave the waitress alone until order or new clients come. This is SUPER efficient.
Async calls can be in multiple things, Database retrievals, Get data from a website, API, etc. It leaves the processor alone until the request is fulfilled.
If you haven’t work with this, you will be totally mesmerized, you will no longer have to wait for a process to finish executing (unless you want to display this info to the user)
PHP is working on multi threads and other stuff what MIGHT work in the future but, the JS Async is just amazing and out of the box
Now that we have covered the basic stuff:
NodeJs is GROWING by the minute while PHP is decreasing but PHP is fighting to stay relevant and frameworks like laravel makes you run an application very fast with a lot of things out of the box, something that JS is not there yet. I have explored some JS frameworks but there are not even so robust as Laravel.
What should I use?
Sorry for the answer, but it really depends on the project. Laravel for me is just WAY easy to use, way more developers (and cheaper than JS devs).
If you want to create something easy, I recommend you to read all the documentation on laravel and understand the basics of an MVC framework.
If you want to make a -request intensive- application I will go with NodeJS (Sails, ExpressJS) but its WAY harder to maintain and not a friendly way to code in an MVC framework.
In my opinion, if you want to create a simple MVP to test the market I would def go with Laravel, it can handle thousands of requests without dropping a sweat (like creating a TODO list for example).
Its important to remember that if your application gains traction you can decouple your application into small units, so your main code can be laravel, and then you can code another section with nodejs so you can have best of both worlds, which is something that I’ve done in the past.
I’ve been very impressed with JS and probably will migrate in the future to only JS ( or a more elegant way to code JavaScript : TypeScript ) but as for now a Hybrid seems to be a great fit for my applications.
I can write more advanced stuff, send me a message about what you want to learn and I will add another post!